01 - Hello World
01 - Hello World
Classroom scripts guide
Each subject discussed during Information Engineering Laboratory classes will be accompanied by a tutorial/script like the one below. Sometimes the tutor will guide you threw the script, sometimes you will be encouraged to read it by yourself and perform parts of the exercise by your own.
In course of the scripts you will encounter blocks marked as:
🔨 🔥 Assignment 🔥 🔨
These are the tasks that you will have to perform during classes. You can always ask the tutor for help or explanation of the assignment. Bare in mind that the teacher can modify the assignments, omit, or add an additional one - so PAY ATTENTION.
Most of the class scripts will finish with:
Final assignments 🔥 🔨
These are the tasks that you will be performing after finishing tutor-guided script. If you do not finish all assignments during the class remember to complete them at home!
Sometimes a homework will be included in the script, marked as:
Homework 💥 🏠
HOMEWORK SOMETIMES CAN BE GRADED!, also your teacher can assign you with additional homework apart from the one included in script.
Used Tools
During this classed a Qt Creator IDE will be our main tool for writing and compiling our programs (https://www.qt.io/). All the tools needed are already installed on laboratory computers. If you wish to install the same set of tools on your home computer see tutorial: 00 - Installing Qt Creator at your home computer.
Additional external tool for code editing and preview, with syntax highlighting can come in handy, choose one you like the most. Recommendations:
- Visual Studio Code (Windows, Linux, OS X) - https://code.visualstudio.com/
- Atom (Windows, Linux, OS X) - https://atom.io/
- Notepad++ (Windows) - https://notepad-plus-plus.org/
- Programmer’s Notepad (Windows) - https://www.pnotepad.org/
- gedit (Linux) - https://wiki.gnome.org/Apps/Gedit
- BBEdit (OS X) - https://www.barebones.com/products/bbedit/
Crating new Qt Creator C++ project
🔨 🔥 Assignment 🔥 🔨
Create a new Qt Creator C++ project using the instruction below
Open Qt Creator, select Welcome(1) tab, than Projects(2) and New Project(3). You can also select File → New File or Project…
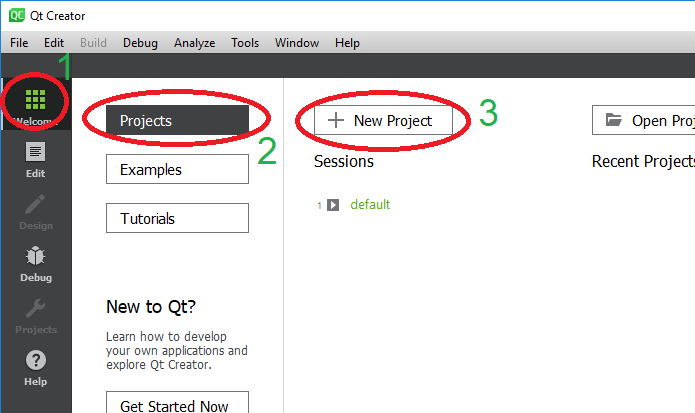
In New Project popup select Non-Qt Project(1), than Plain C++ Application(2) and Choose(3):
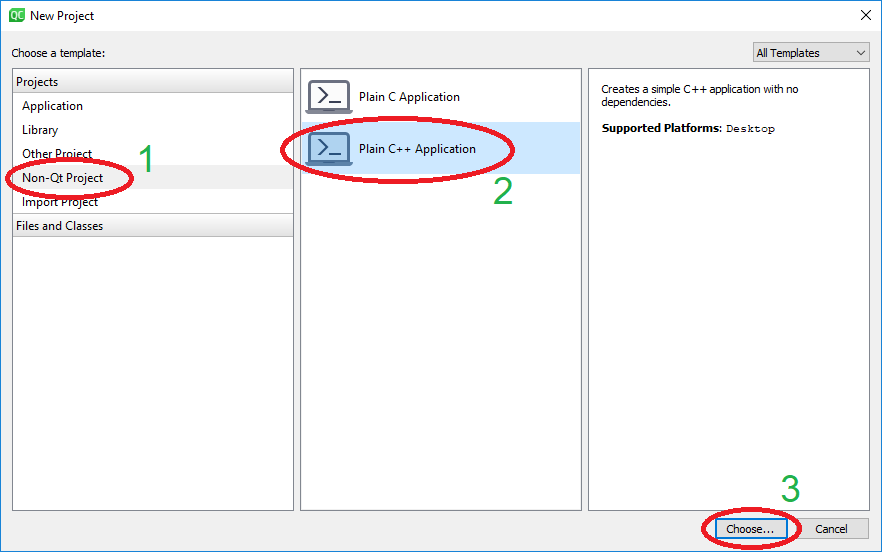
Next we have to name the project(1) - hello_world in this case. You can also change the destination folder. CAUTION: In project names and localization do NOT use special signs (like polish letters) and additional spaces (use underscore if needed). Select Next(2).
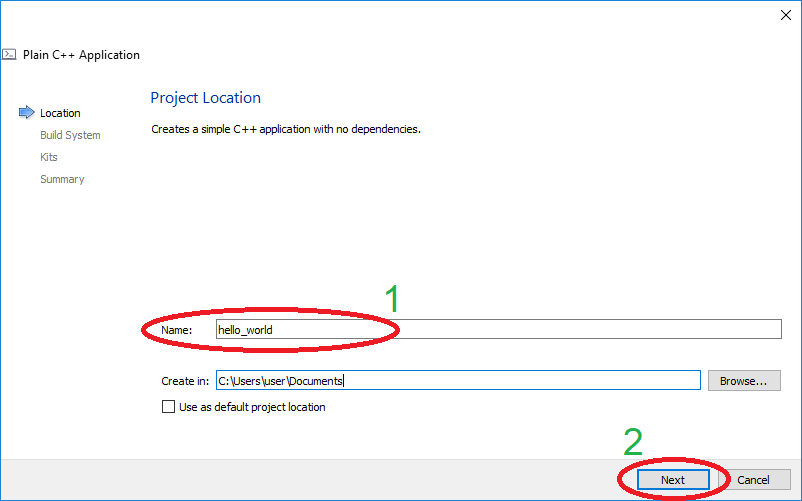
Now you have to select a Build System - it is a set of tool that will be responsible for preparing a project for each compilation process. While working with Qt it is the easiest to use qmake. Make sure it is selected(1), and click Next(2).
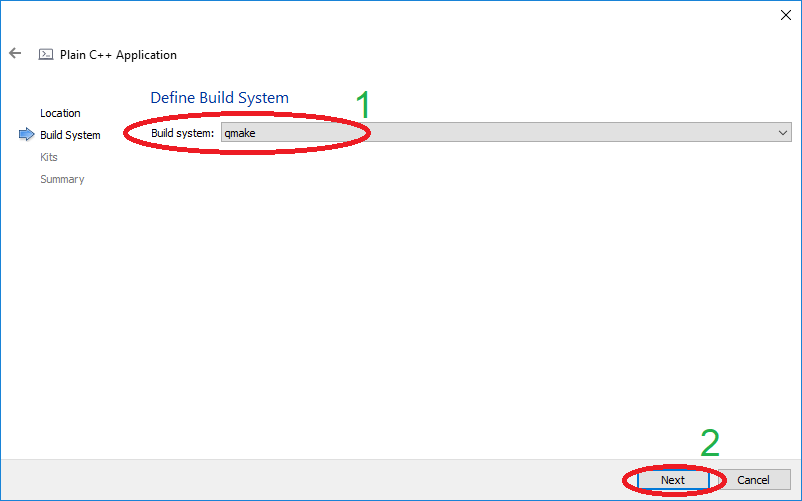
Next wizard window can differ on different machines. In this step you will be choosing a set of compiler and debugger tools that will be used in created project. During our classes we will be using MinGW 64-bit. Make sure that this, and only this one, kit is selected by ticking the box left to the kit name(1). At home also use MinGW 64-bit in order to avoid incompatibilities. Click Next(2).
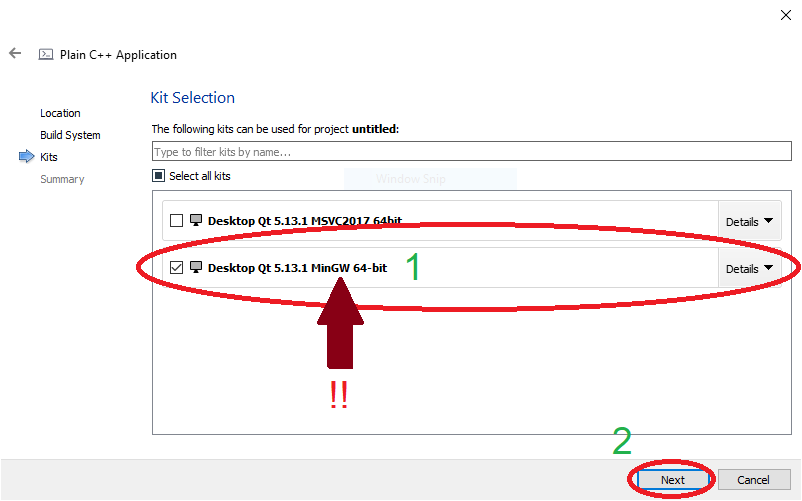
On the last step just hit Finish.
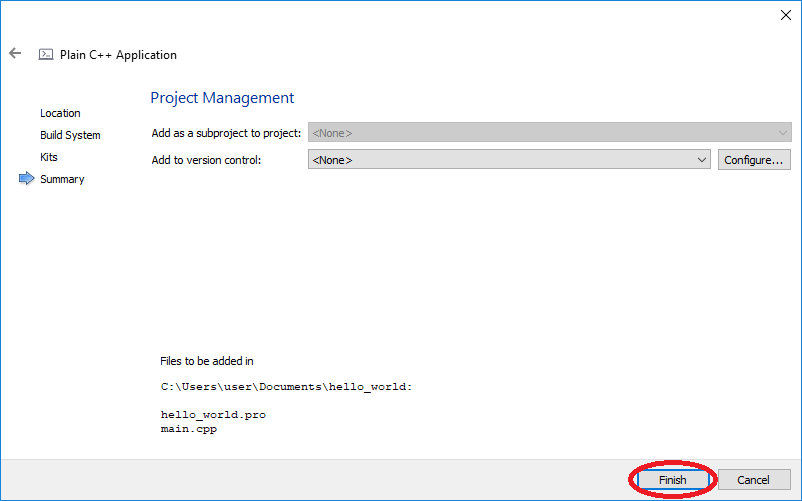
First Program - Hello World!
Qt Creator will always create a Hello World program:
#include <iostream>
using namespace std;
int main()
{
<< "Hello World!" << endl;
cout return 0;
}
Entry function int main()
is usually placed in
main.cpp file - see a project tree.
In order to build the program select a hammer icon or hit a keyboard
combination: Ctrl+B. If a compilation process is a success you
will see a green progress bar in right corner to fill completely:
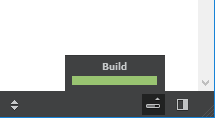
To run the program click the play button: or hit a keyboard
combination: Ctrl+R. A comment window displaying “Hello World!”
should pop up.
🔨 🔥 Assignment 🔥 🔨
Build and run the “Hello World!” program.
Commenting code
C++ offers two types of comment styles:
- one-line comments:
// can be used to temporarily deactivate a line of code
// cout << "Hello World!" << endl;
// or can be used to add a line description
<< "Hello World!" << endl; // prints "Hello World!" and a new line cout
- multi-line comments:
/*
used primarely
for longer comments
*/
/* can also be used to deactivate a block of code
cout << "Hello World!" << endl;
return 0;
*/
ATTENTION Comment your code! It will help you to understand what each line means. It is always easier to understand commented code on return after few days!
🔨 🔥 Assignment 🔥 🔨
Try inserting one-line and multi-line comments into the code.
Deactivate line cout << "Hello World!" << endl;
using both types of comment, re-build the program and run. What is the
result?
Add one-line comments describing selected lines.
Add multi-line comment:
/*
The main function is
a starting point of application
*/
above int main()
line.
Code errors handling
In order for program to work a syntax of the written code have to be
strict with a guidelines of particular programming language. While
writing a code Qt Creator will highlight potential errors in our code.
Eg. in C++ each instruction have to be ended with ;
. After
deleting the semicolon, after few seconds, editor will highlight this
line red and suggest potential mistake:

🔨 🔥 Assignment 🔥 🔨
Imitate various code errors, read the error comment suggested by Qt Creator, fix it back and try another one:
- delete
;
(semicolon) from any line in the code - delete
}
(curly bracket) from the last line of the code - modify
int
tointa
from lineint main()
- add
foo = 1;
in line belowcout << "Hello World!" << endl;
If one tries to build the program containing errors Qt Creator will not compile it. Issues tab will be opened with a list of potential errors. You can double click any of the raised errors for editor to jump to a faulty line. Try compiling the code containing errors.
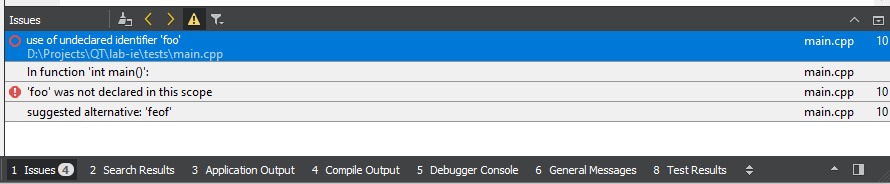
ATTENTION It is very important to read the errors! Most of the mistakes in code can be easily fixed by reading the error message and applying the suggested solution.
Final assignments 🔥 🔨
1. Easy code manipulation
- Try changing the code in a way that it displays more than one “Hello World!” message:
Hello World!
Hello World!
Hello World!
Hello World!
- Modify the displayed text:
My name is AAA BBB
I am from CCC
I am YYY years old
My hobby is ZZZ
- Modify the code so each line is separated by additional line:
Hello World!
Hello World!
Hello World!
Hello World!
2. Install Qt Creator at your home computer
Authors: Tomasz Mańkowski